States
Tracking website interactions made easy is the goal of JENTIS. Here a rather abstract but very helpful concept comes into place: States. Every event can be a State and with each JENTIS takes a snapshot of all properties and variables so you can retrace each data point. So all tracking with JENTIS will stay in contact with a State, as all Tags, Triggers and Variables all work in some context to a State.
The JENTIS States concept creates a uniform scope to work with data server side. As during data computation (evaluating server side variables, executing tags on triggers, etc) the server can not access data from the client (website visitors browser) conveniently.
Users interacting with your website will open the website, wait for it to load, click somewhere and navigate. Generally speaking all websites are built differently, it can be a single page application or a classic link-per-link navigating website. Now it is up to you to use the core web protocols (such as “DOM Ready” or “Page Load”) events and define default JENTIS States or create your own definitions. In any case: JENTIS comes with a lot of built in definitions, so technical skills are not mandatory.
Here is a basic guide to dive deep into this concept: JENTIS States Framework
If you are more into the use cases, here are some guides for you to get started:
Listen to (GTM) Data Layer Events: Listen to Datalayer Events
Clicks, Form Submits and Scroll Tracking: Form Submit, Click and Other Actions
History Change States (Virtual Pageviews): History Change State and Virtual Pageviews
State Registry - Creating and Managing JENTIS States
Creating and customizing a state can be achieved in your JTM account. Navigate to the Server Tag Manager: States area to edit or create new State objects. Here you can edit existing State definitions or create new ones.
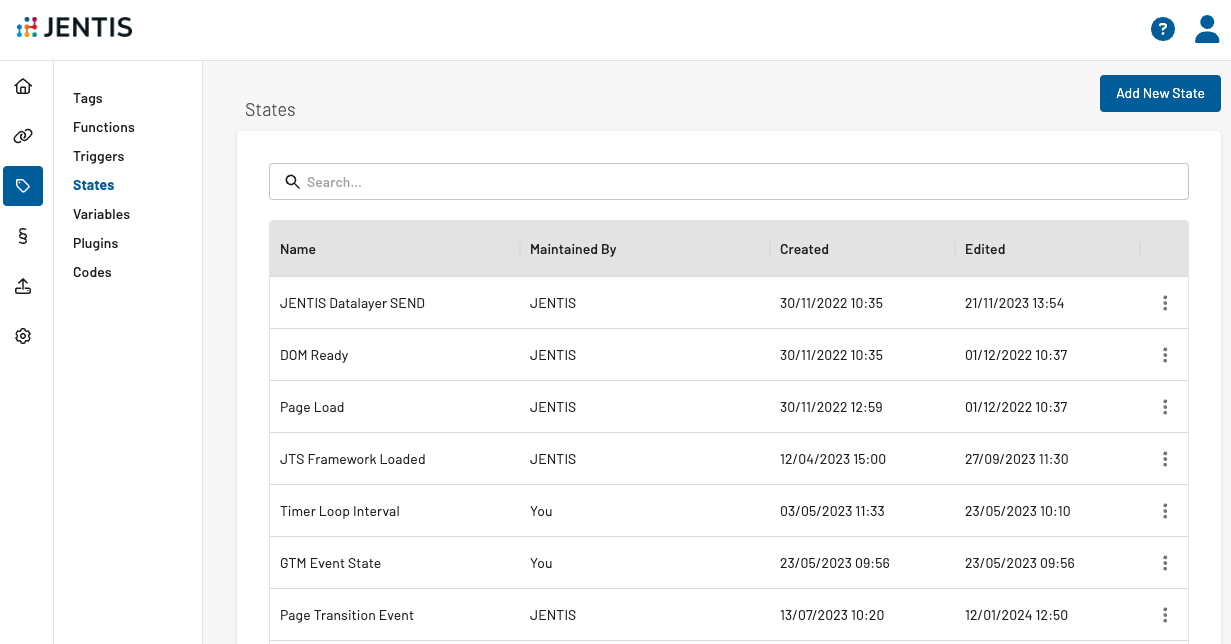
A States has the basic parameters such as name, description or id, as with all elements in JENTIS. The JavaScript code will define a function with a single input parameter (a function) and must call the input parameter at the end. The following example will help you to better understand this concept.
State Function Reference
A state is an anonymous JavaScript function with one input parameter. This function will determine the event (a moment in time or interaction of a client) and submit a callback.
Anonymous Function Input Parameter: {function} initState
The state code statement must begin with an anonymous function with an input object. That input is a callback function and must be called whenever this JENTIS State is determined to be activated.
Further the initState (callback function) takes an Object as the input with certain properties. This object and its properties is optional and only for advanced users.
initState input Object properties:
stateCallback: if you want to call a function after this JENTIS State was processed this is the place to go.
contextualStateInformation: this is a customizable Object that you can submit when this JENTIS State is processed and access this properties and values in JENTIS Frontend Variables.
For the usage of this advanced settings please see in the section below for a detailed use case running through this options.
Basic State Definition – DOM Ready State
The most basic use case for a state is the DOMContentLoaded event handle. This will create a JENTIS State in your JTM account that activates on the named web browsers event. Further details on this api and specs can be found here: DOMContentLoaded event – Web APIs | MDN
DOM Ready State JavaScript Function
function(initState) {
document.addEventListener('DOMContentLoaded', (event) => {
initState();
});
};
With this state in place your JTM will react as soon as the event was triggered in a users browser. Activating all triggers, checking conditions and calculating all Frontend Variables values. So with this state you are ready to go, if this event fits your requirements.
Advanced State Use Case
In some cases it is important to further customize a JENTIS State. With the input to the initState function you can do so with the optional properties documented above.
Let's run through an example that generates a more enhanced JENTIS State. Here we will activate a JENTIS State for each external data layer item, where we must process each item individually and not from a global perspective.
This is important in situations where JENTIS is loaded asynchronously and accesses a pre existing data layer. The following might be a simple precondition.
var dataLayer = [{
event: "1"
name: "event_1"
},{
event: "2",
name: "event_2"
}];
If such a dataLayer object is defined before JENTIS is loaded we must be sure to process each of this events individually. As from a global perspective there is no certain "event" but multiple entries. Which might bring us into trouble, if we'd like to activate a trigger on the event name "event_1". As the array contains two such entries and we might miss either of both if we not check each event individually as a JENTIS State.
To resolve this the contextualStateInformation comes into play. For each JENTIS State an object with a snapshot of an event can be submitted. Let's give this a try:
function(initState){
//generate this object with the mentioned properties in an appropriate scope
optionalParamObj = {};
//resetting the JENTIS Data Layer is optional in this case, as no further information is pushed this time
optionalParamObj.stateCallback = function() {
window.jentis.tracker.resetDatalayer();
}
for(var i = 0; i < dataLayer.length; i++){
optionalParamObj.contextualStateInformation = dataLayer[i];
initState(optionalParamObj);
}
}
With this example we execute each entry of the dataLayer (presumably that is an event object). Now we can be sure each such external events is translated into a JENTIS State without losing the context (event object).
Now this all would be fruitless if no element takes advantage of the contextual object. This can be accessed in frontend variables (custom javascript variable - Variables).
You can create such a variable and access from a JENTIS State the according object. Which might be the events "name" value.
function(stateContextObject){
if(stateContextObject.stateContext &&
stateContextObject.stateContext.name)
return stateContextObject.stateContext.name;
else
return undefined;
}
This will return the "name" property of the event (from the dataLayer object submitted in the JENTIS State code above).
Now with this functionality you can build and customize your JENTIS Tag Manager configuration around all kinds of use cases. Please let us know if you find it useful and what kind of use cases you are working with.